AWS SDK for PHPでS3を操作する
今回は、PHP用のSDKである「AWS SDK for PHP」でS3バケットを操作してみます。
SDKのインストール
SDKはComposerを使ってインストールができます。GitHubからソースをダウンロードして配置することも可能です。
今回はComposerを使ってインストールしてみます。
コマンドプロンプトで下記のようにコマンドを実行します。
>composer require aws/aws-sdk-php
Using version ^3.100 for aws/aws-sdk-php
./composer.json has been created
Loading composer repositories with package information
Updating dependencies (including require-dev)
Package operations: 7 installs, 0 updates, 0 removals
- Installing mtdowling/jmespath.php (2.4.0): Downloading (100%)
- Installing guzzlehttps/promises (v1.3.1): Loading from cache
- Installing ralouphie/getallheaders (2.0.5): Loading from cache
- Installing psr/https-message (1.0.1): Loading from cache
- Installing guzzlehttps/psr7 (1.5.2): Loading from cache
- Installing guzzlehttps/guzzle (6.3.3): Loading from cache
- Installing aws/aws-sdk-php (3.100.9): Downloading (100%)
guzzlehttps/guzzle suggests installing psr/log (Required for using the Log middleware)
aws/aws-sdk-php suggests installing ext-sockets (To use client-side monitoring)
aws/aws-sdk-php suggests installing doctrine/cache (To use the DoctrineCacheAdapter)
aws/aws-sdk-php suggests installing aws/aws-php-sns-message-validator (To validate incoming SNS notifications)
Writing lock file
Generating autoload files
vendor/autoload.php
をrequireすればSDKを使用することができます。簡単ですね。
S3にファイルをアップロード
では実際にコードを書いてS3にファイルをアップロードしてみます。ComposerでSDKをインストールしたディレクトリに
upload.php
を作成しました。<?php
// SDKの読み込み
require 'vendor/autoload.php';
use Aws\S3\S3Client;
// S3設定ファイルの読み込み
$array_ini_file = parse_ini_file('credentials.ini', true);
// S3Clientインスタンスの作成
$s3client = S3Client::factory([
'credentials' => [
'key' => $array_ini_file['ACCESS_KEY'],
'secret' => $array_ini_file['SECRET_ACCESS_KEY'],
],
'region' => 'ap-northeast-1',
'version' => 'latest',
]);
// ローカルにファイル作成
$file = date('YmdHis') . '.txt';
$content = <<< EOF
hoge
ふが
EOF;
file_put_contents($file, $content);
// S3にアップロード
$result = $s3client->putObject([
'ACL' => 'public-read', // ACLを指定する場合、ブロックパブリックアクセスはすべてオフにする
'Bucket' => $array_ini_file['BUCKET_NAME'],
'Key' => 'test.txt',
'SourceFile' => $file,
'ContentType' => mime_content_type($file),
]);
// 結果を表示
echo('<pre>');
var_dump($result);
echo('</pre>');
IAMユーザーのクレデンシャルとバケット名は同一の階層にcredentials.iniというファイルを作って外出ししています。
これを実行すると…
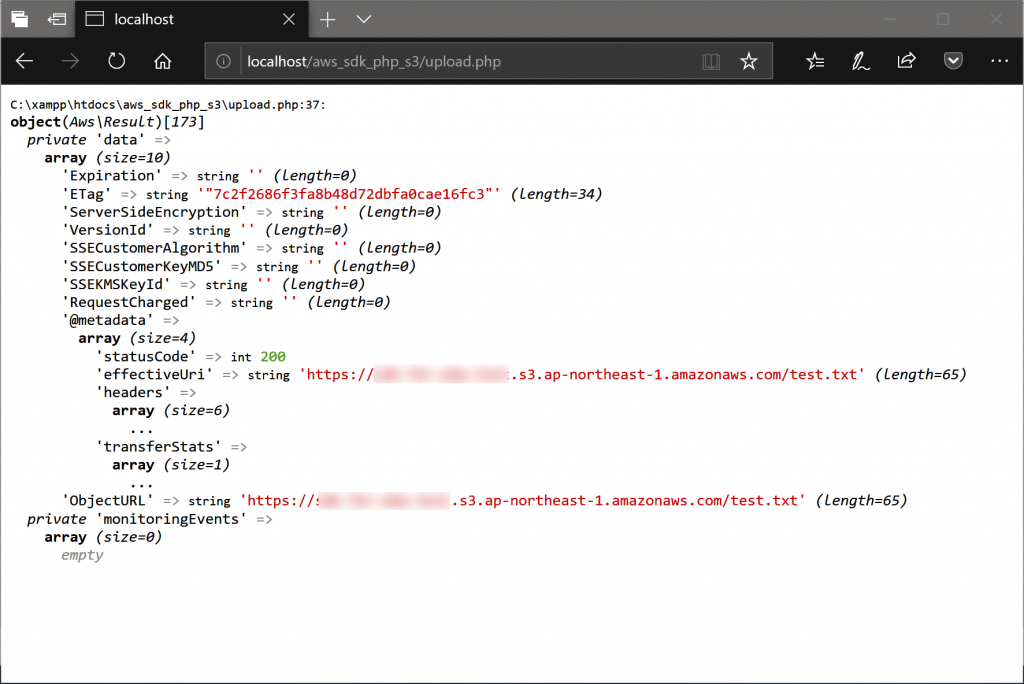
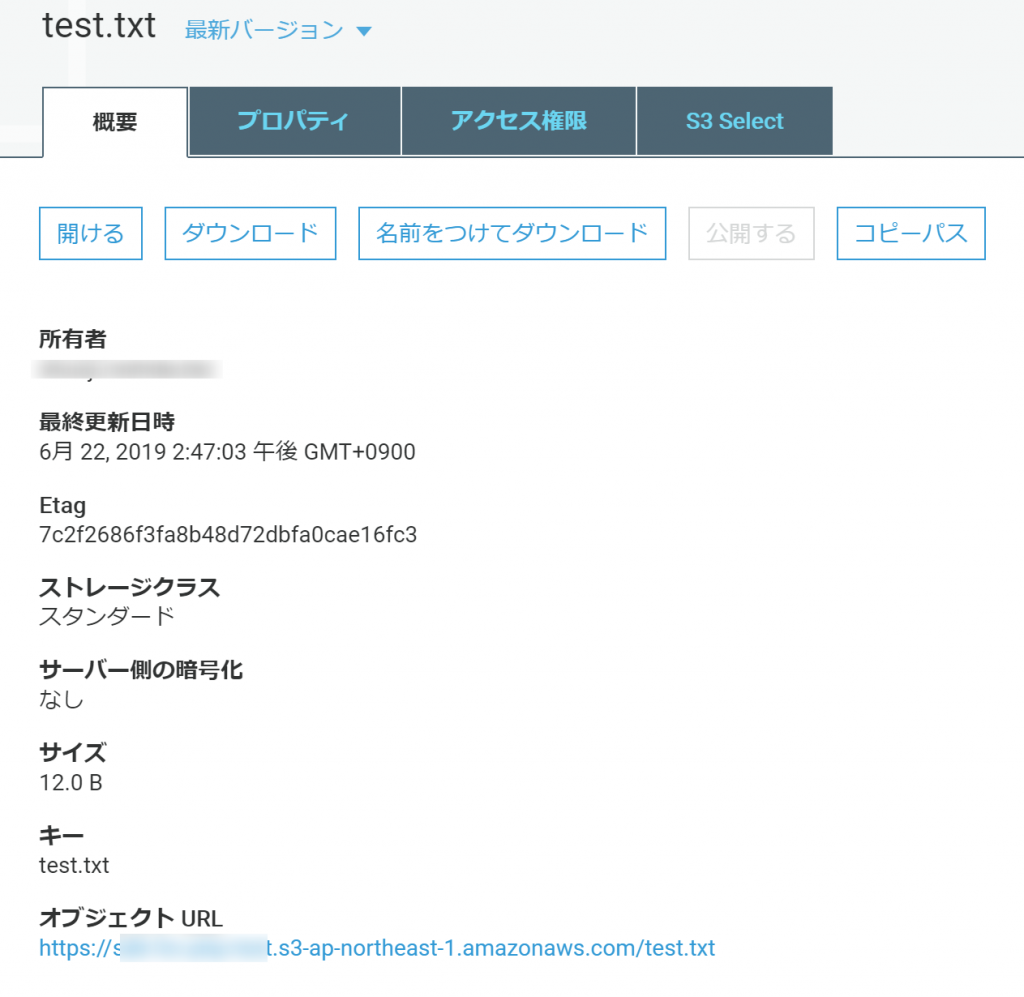
ちゃんとS3にファイルがアップロードされました!
S3からファイルをダウンロード
今度は、S3にアップロードしたファイルをローカルにダウンロードしてみます。同じ階層に今度は
download.php
を作成します。<?php
// SDKの読み込み
require 'vendor/autoload.php';
use Aws\S3\S3Client;
// S3設定ファイルの読み込み
$array_ini_file = parse_ini_file('credentials.ini', true);
// S3Clientインスタンスの作成
$s3client = S3Client::factory([
'credentials' => [
'key' => $array_ini_file['ACCESS_KEY'],
'secret' => $array_ini_file['SECRET_ACCESS_KEY'],
],
'region' => 'ap-northeast-1',
'version' => 'latest',
]);
// S3からダウンロード
$result = $s3client->getObject([
'Bucket' => $array_ini_file['BUCKET_NAME'],
'Key' => 'test.txt',
]);
// 結果を表示
header("Content-Type: {$result['ContentType']}");
echo $result['Body'];
ダウンロードしたファイルの情報は
$result
に格納されています。これをファイルに書き出して保存することもできますし、そのままレスポンスに出力することもできます。
今回はレスポンスに出力しています。
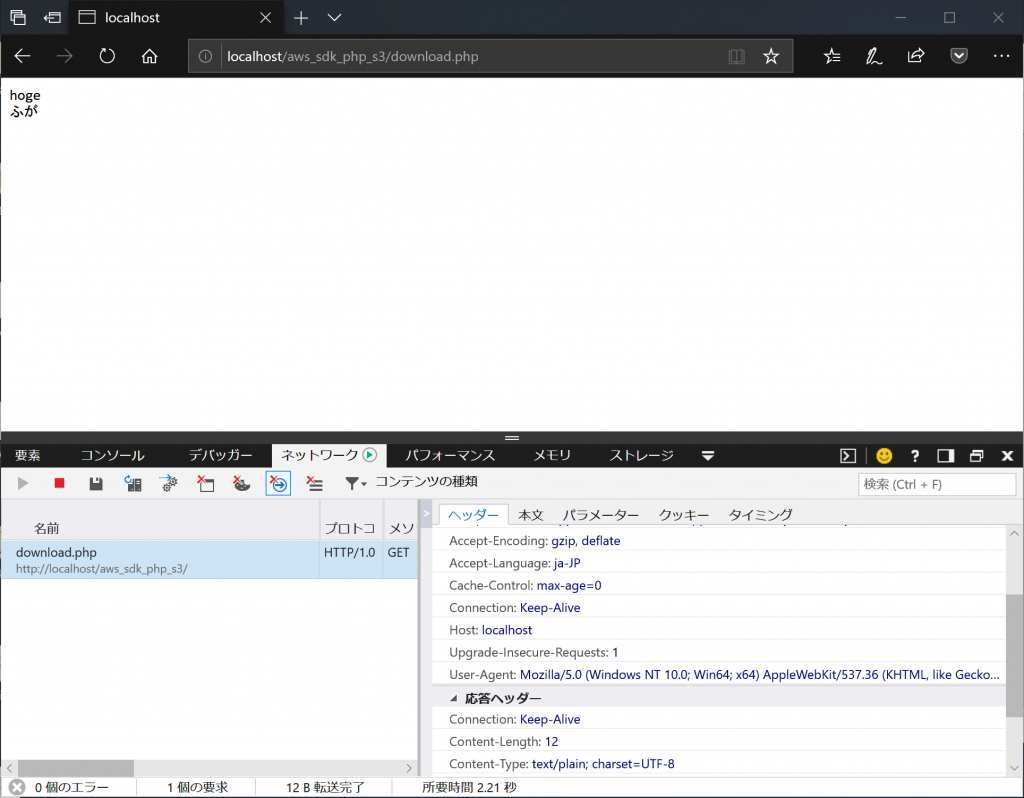
アップロードしたファイルの内容が出力されています!
思った以上に簡単にS3へのアップロードとダウンロードが実装できました。
もっとSDKを使いこなしてAWSのサービスを生かしたWebアプリを作ってみたいなー。
ディスカッション
コメント一覧
まだ、コメントがありません